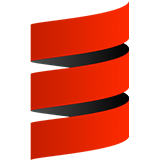
JVM Drivers
Fauna’s open source JVM driver supports languages that run in the Java Virtual Machine. Currently, Java and Scala clients are implemented.
Features
-
All drivers fully support the current version of the FQL API.
-
Java and Scala clients share the same underlying library faunadb-common.
-
Supports Dropwizard Metrics hooks for stats reporting.
Documentation
Javadocs and Scaladocs are hosted on GitHub:
Detailed documentation is available for these languages:
Dependencies
Shared
-
Jackson for JSON parsing.
-
Async HTTP client and Netty for the HTTP transport.
-
Joda Time for date and time manipulation.
Install
Usage
Java
import com.faunadb.client.FaunaClient;
import static com.faunadb.client.query.Language.*;
/**
* This example connects to FaunaDB using the secret provided
* and creates a new database named "my-first-database"
*/
public class Main {
public static void main(String[] args) throws Exception {
//Create an admin connection to FaunaDB.
FaunaClient adminClient =
FaunaClient.builder()
.withSecret("put-your-key-secret-here")
.build();
adminClient.query(
CreateDatabase(
Obj("name", Value("my-first-database"))
)
).get();
client.close();
}
}
Scala
import faunadb._
import faunadb.query._
import scala.concurrent._
import scala.concurrent.duration._
/**
* This example connects to FaunaDB using the secret provided
* and creates a new database named "my-first-database"
*/
object Main extends App {
import ExecutionContext.Implicits._
val client = FaunaClient(
secret = "put-your-secret-here"
)
val result = client.query(
CreateDatabase(
Obj("name" -> "my-first-database")
)
)
Await.result(result, Duration.Inf)
client.close()
}
Next steps
-
Driver repository: https://github.com/fauna/faunadb-jvm
-
For more information about the Fauna Query Language, consult our query language reference documentation.
Was this article helpful?
We're sorry to hear that.
Tell us how we can improve!
documentation@fauna.com
Thank you for your feedback!